I was developing an app on Microsoft Power Platform for internal use and came across a use case which appeared quite simple at first, but a bit complicated to implement within a Power Automate flow.
Every day, I would be provided a list of employees that are available on that day for a brief day-end meeting, discussing how their day went. The employees needed to be paired randomly in a group of two people. In case there is an odd number of participants, one pair needed to include 3 people so that we don’t end up with a loner. Then MS Teams meeting invites needed to be sent to them all.
Designing the solution seems quite straightforward by utilizing Power Automate actions and storing/retrieving information from Dataverse. However, I got stuck at the randomizing part, since I couldn’t find a built-in function or an action that could do that. I did some research and found out that most people have been using Azure functions to handle such cases, my use case differed from the solutions out there, so I had to build a function of my own.
I am quite familiar with Python and have used it in the past for various data related tasks. Therefore, I decided to use Python here as well to set up a function with relevant logic. Azure Function Apps are very easy to setup, you can have one up and running within 5 minutes.
Following steps will guide you how to create Azure Function App using Azure Portal.
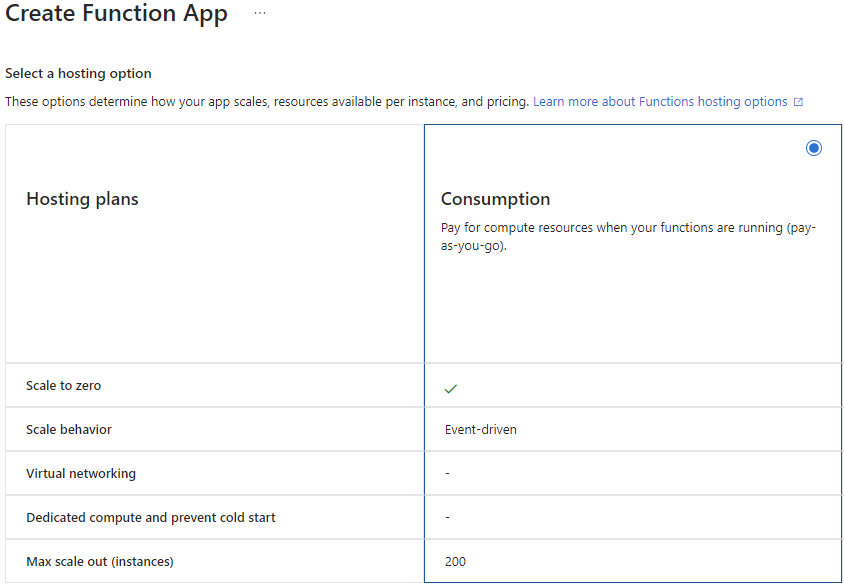
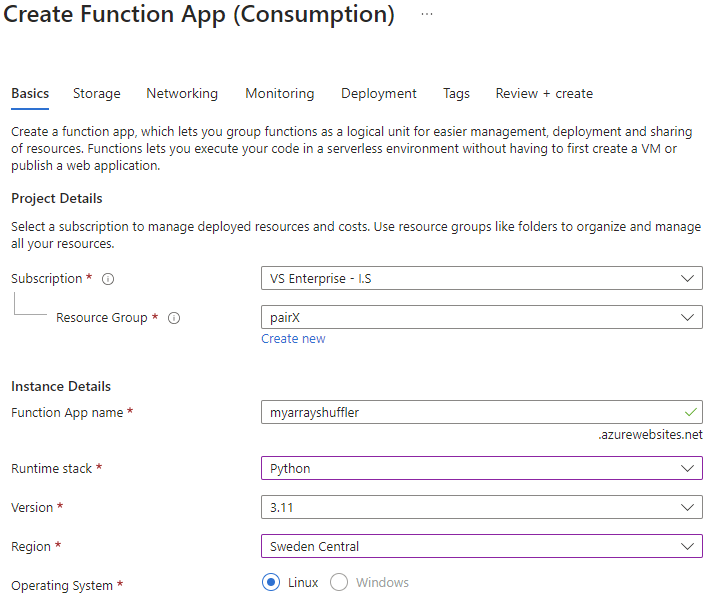
Choosing the Create in Azure portal is the simplest method, you can test it directly inside the portal.
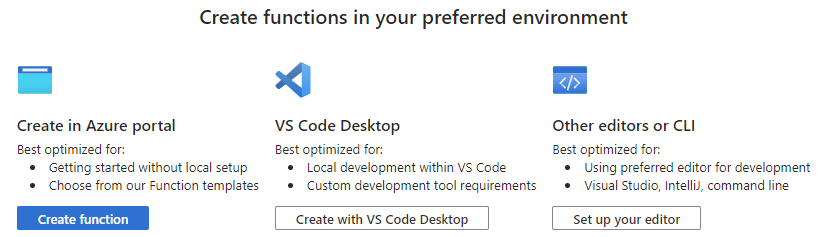
Since we will be using an HTTP action in power automate to send our data, we need to choose HTTP trigger template.
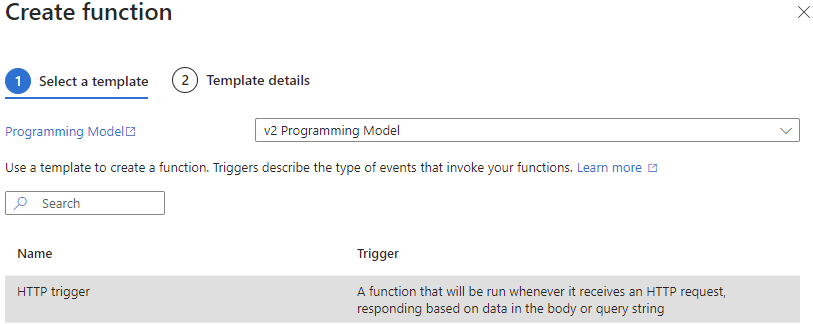
Provide a name for your function
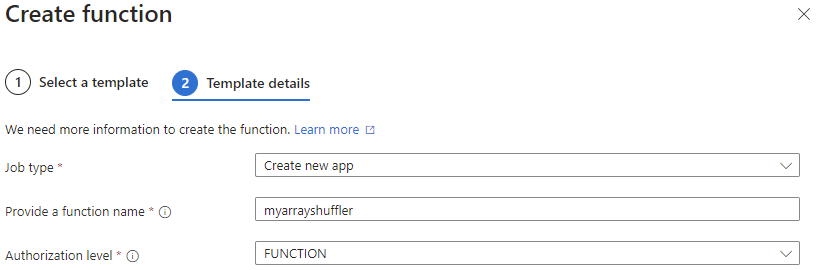
Use the Test/Run screen to enter test data.
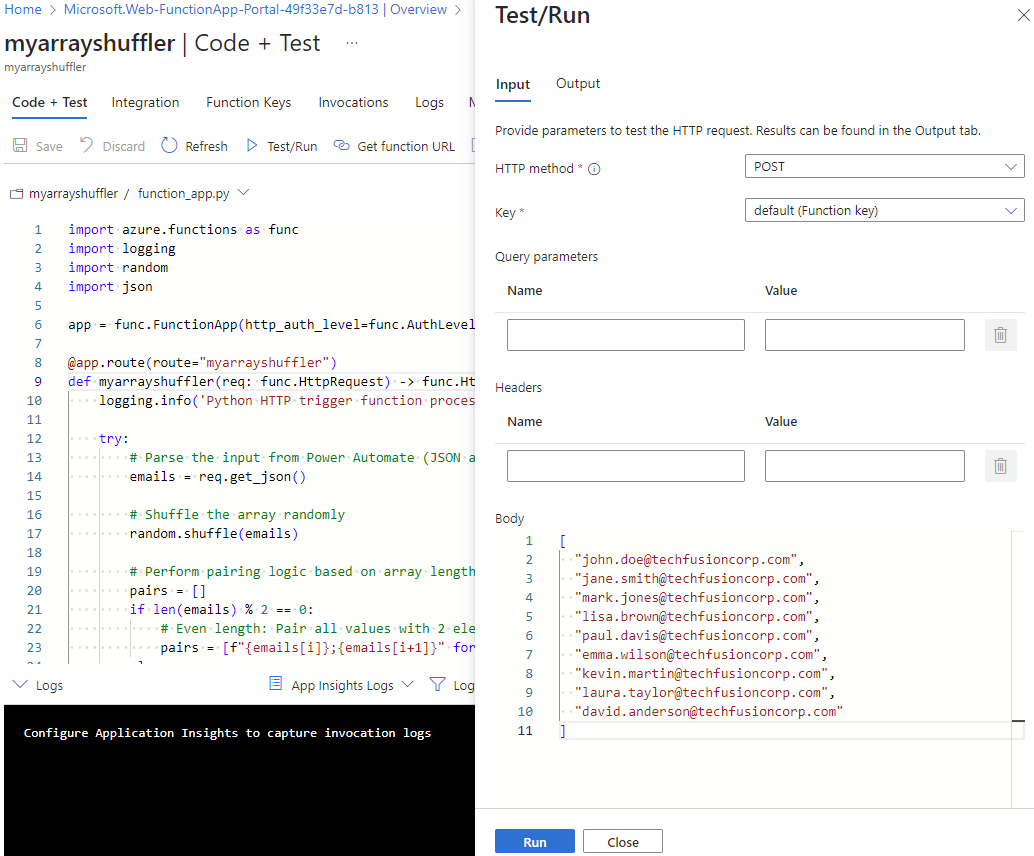
From the outputs tab, verify the outputs of test data.
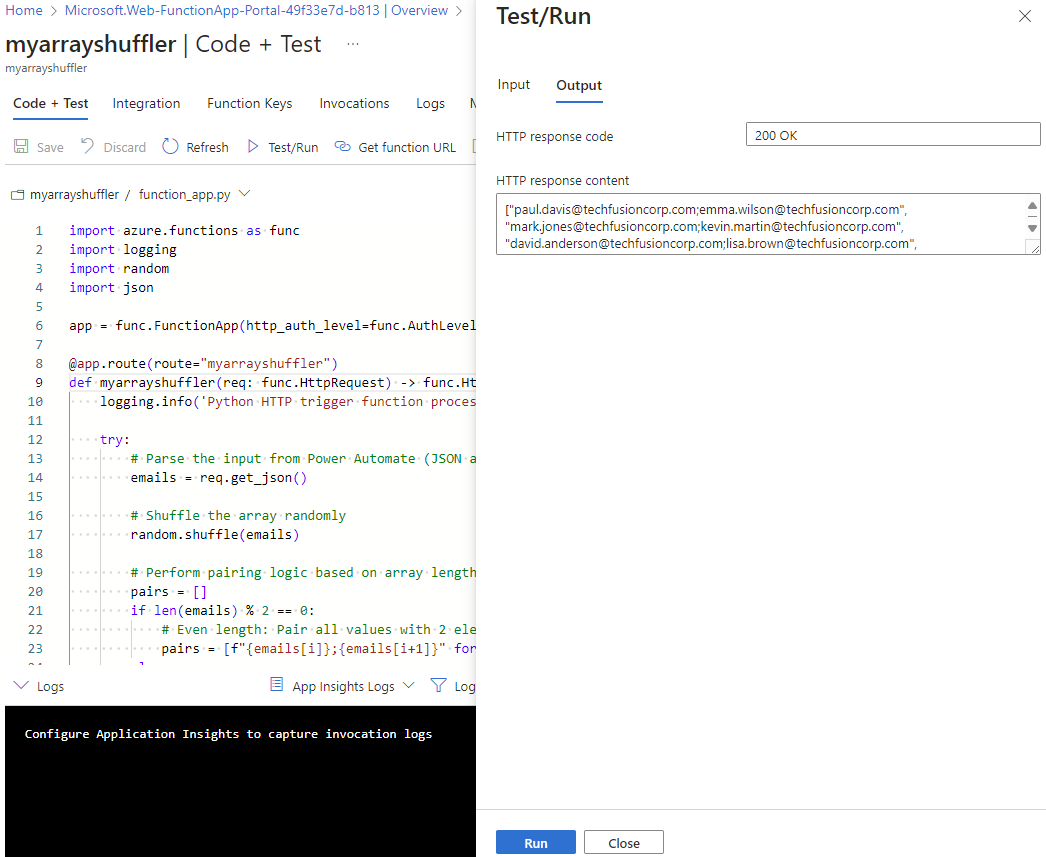
Get the function URL which will be required to make a call from HTTP action inside power automate. Use the default (Function key)
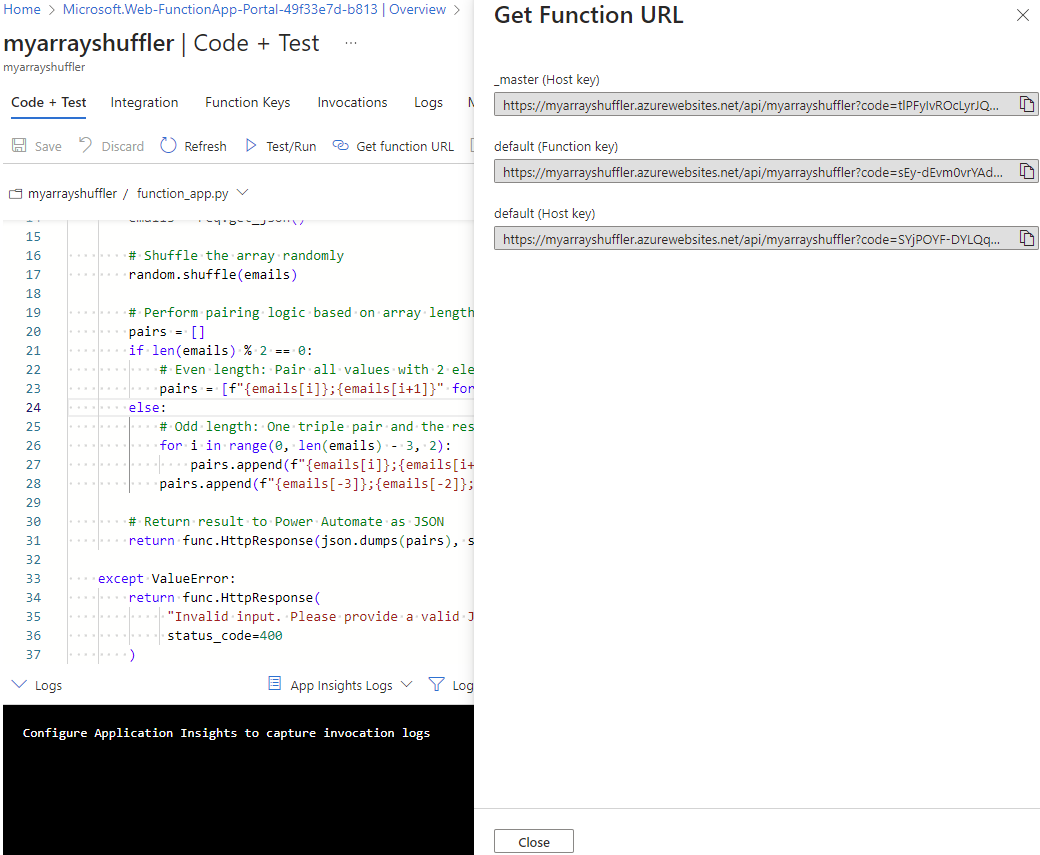
The random.shuffle() functions takes an input array and shuffles it randomly, then I simply used for loop to append the elements of array next to each other into my pairing array. This array is then returned by the function and can be used in the next actions in Power Automate.
The code in Azure function is as follows:
import azure.functions as func
import logging
import random
import json
app = func.FunctionApp(http_auth_level=func.AuthLevel.FUNCTION)
@app.route(route="myarrayshuffler")
def myarrayshuffler(req: func.HttpRequest) -> func.HttpResponse:
logging.info('Python HTTP trigger function processed a request.')
try:
# Parse the input from Power Automate (JSON array)
emails = req.get_json()
# Shuffle the array randomly
random.shuffle(emails)
# Perform pairing logic based on array length
pairs = []
if len(emails) % 2 == 0:
# Even length: Pair all values with 2 elements
pairs = [f"{emails[i]};{emails[i+1]}" for i in range(0, len(emails), 2)]
else:
# Odd length: One triple pair and the rest with 2 elements
for i in range(0, len(emails) - 3, 2):
pairs.append(f"{emails[i]};{emails[i+1]}")
pairs.append(f"{emails[-3]};{emails[-2]};{emails[-1]}") # Last 3 values as a triple pair
# Return result to Power Automate as JSON
return func.HttpResponse(json.dumps(pairs), status_code=200)
except ValueError:
return func.HttpResponse(
"Invalid input. Please provide a valid JSON array of emails.",
status_code=400
)
Now, moving towards Power Automate flow, create a scheduled flow and incorporate necessary actions as following.
Initialize an Array variable, this will hold our email addresses. The email addresses come from a Dataverse table. You can use any source of your choosing.
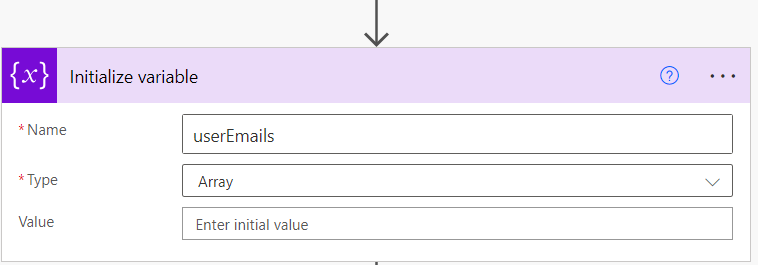
We iterate through the rows that we get from List Rows action and store email addresses in our userEmails array.
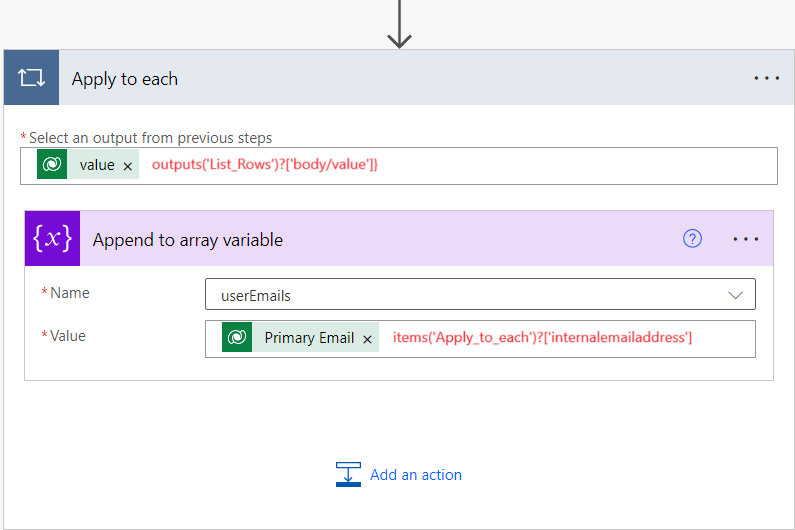
After this action, the userEmails looks like as follows:
[
"john.doe@techfusioncorp.com",
"jane.smith@techfusioncorp.com",
"mark.jones@techfusioncorp.com",
"lisa.brown@techfusioncorp.com",
"paul.davis@techfusioncorp.com",
"emma.wilson@techfusioncorp.com",
"kevin.martin@techfusioncorp.com",
"laura.taylor@techfusioncorp.com",
"david.anderson@techfusioncorp.com"
]
We then use an HTTP action to call our function. We should use POST method and the URI from figure 8. We also need to pass our userEmail array in the Body.
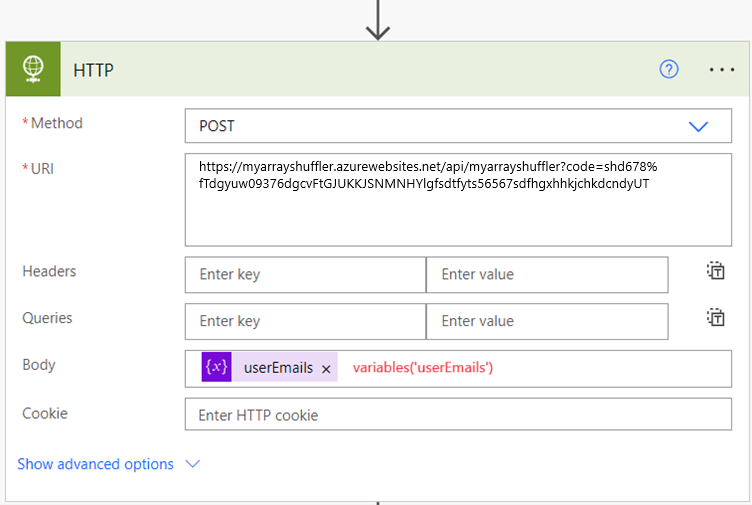
The output from HTTP action looks like following. Exactly the way we saw in our testing. It is of type string.
[ "john.doe@techfusioncorp.com;mark.jones@techfusioncorp.com", "lisa.brown@techfusioncorp.com;david.anderson@techfusioncorp.com", "paul.davis@techfusioncorp.com;emma.wilson@techfusioncorp.com", "jane.smith@techfusioncorp.com;kevin.martin@techfusioncorp.com;laura.taylor@techfusioncorp.com" ]
We then convert the string output from HTTP action into an array myArr
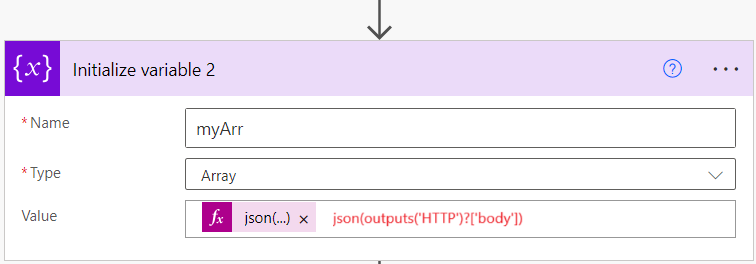
Having the output delimited as semi-colon is required because they can be directly used in the Create a Teams meeting action. The array myArr looks like:
[
"john.doe@techfusioncorp.com;mark.jones@techfusioncorp.com",
"lisa.brown@techfusioncorp.com;david.anderson@techfusioncorp.com",
"paul.davis@techfusioncorp.com;emma.wilson@techfusioncorp.com",
"jane.smith@techfusioncorp.com;kevin.martin@techfusioncorp.com;laura.taylor@techfusioncorp.com"
]
The elements of the array myArr are then used directly in the Required attendees
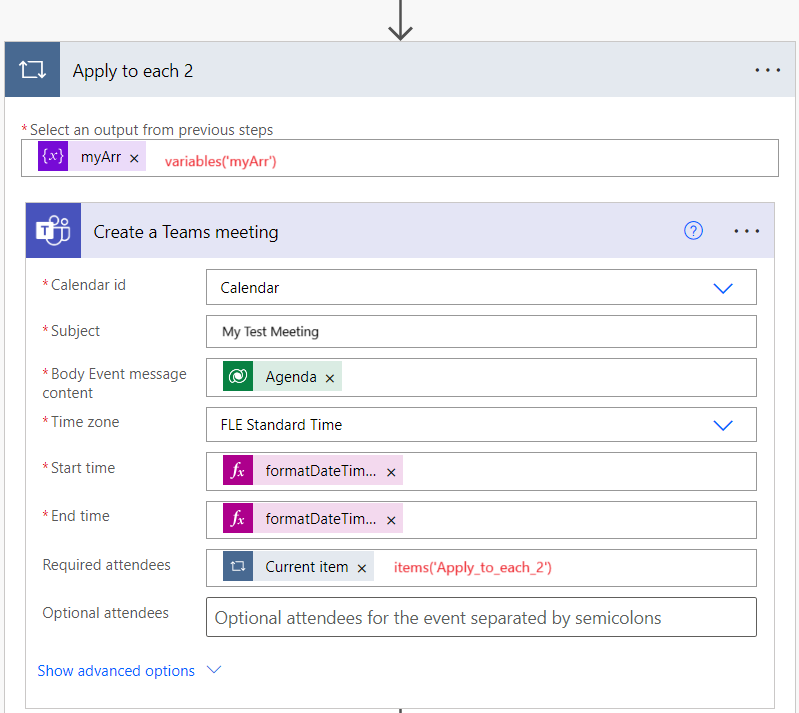
And that's how you can combine Power Automate Flow with Azure Functions. This automation was really fun to build and of course made my work a lot easier. I hope you found it useful.
Liked this post? Follow Bofor on LinkedIn to get the latest updates.
Contact us to learn more about Bofor and what we can do for you.